Lazy Loading with EF Core Proxies
With the Microsoft.EntityFrameworkCore.Proxies NuGet package you can use to traverse navigation properties. This is often preferable to joins and includes such as when using one-to-many or only exploring a subset of the navigations based on client-side logic or for providers that don't support include yet.
ZX Gona

Gona is an incredibly popular typeface used in Japan from everything from signage to packaging to user interfaces. It's a Latin typeface that is even more popular than Helvetica is in the western world. It's a clean, smart sans-serif that is very easy to read.
Doublewear

Yugoslavian designer Vinko Ožić Pajić produced a logo designs for the Standard Konfekcija company in 1963 with this very unique and distinctive style.
Wand

A brand new all-original condensed italic/oblique with a slight curvature and flourishes gives a nice smooth and yet modern feel to anything set it in - even in so few pixels.
ZX Amelia

Stan Davis' 1964 typeface Amelia takes inspiration from the magnetic OCR of its time but in a friendly, rounded & bold style.
Nuxt Content v1 content.db database & file size
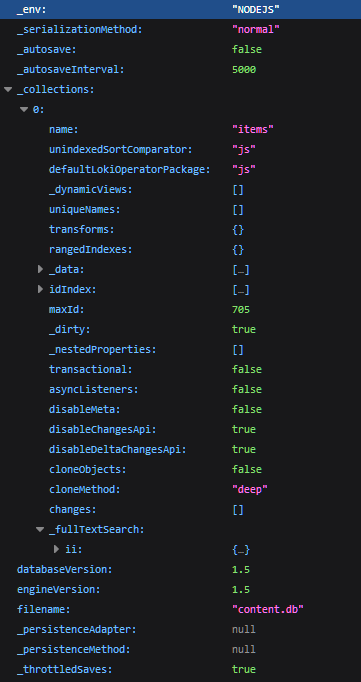
An examination of Nuxt's content.db and how to shrink it for performance.
Melanie

I created this stylish new 8x8 bitmap font after being inspired by a recent fully-scalable typeface called Melindrosa by Flavia Zimbardi - if you're looking for a gorgeous new art deco font you should definitely check it out.
Hyperlight

A new futuristic open face pruned back to the basics with isolated segments allowing them to be easily stencilled on the side of a space, air, land or sea craft - or just to save time etching or spraying.
ZX Revue

Revue, created by Colin Brignall in 1968, could be found everywhere in the 70s & 80s. Gamers might recognize it from the title screen and livery of the 1987 arcade classic After Burner from SEGA.
Bitty

Narrow glyphs are common on the Speccy with 64-column routines gracing Your Sinclair as well as word-processors at the cost of readability.
Coded Entry

The blobs on fonts were to allow optical or magnetic ink readers to quickly scan documents - many over a certain age will remember them from the bottom of cheques.
Email form sender with Nuxt3, Cloudflare, Brevo & reCAPTCHA
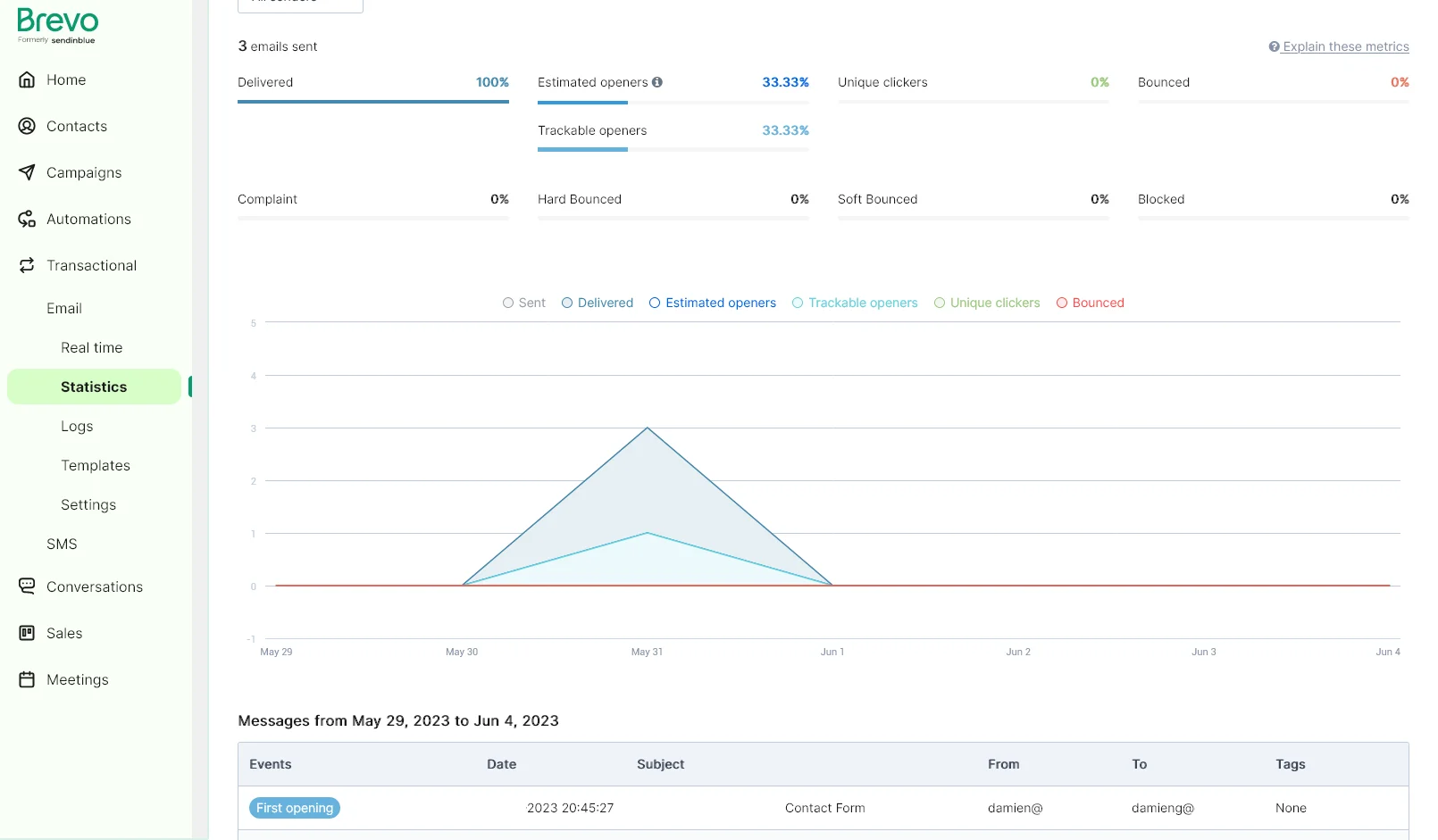
I've been using Nuxt quite extensively on the static sites I work on and host and use Cloudflare Pages to host them. It's a great combination of power, flexibility, performance and cost (free).
Rendering content with Nuxt3
I've been a big fan of Nuxt2 and Nuxt3 is definitely a learning curve and I have to admit the documentation is a bit lacking - lots of small fragments and many different ways to do things.
Pixie

Here's the ultimate day of my 2022 Advent of Fonts - a family of small fonts called Pixie. Included are the usual bold variant but also a squared-off digital and then a bell-bottomed one and even a stretched out top variant of that for a little unusual variety.
Treatise

Day 19 of the 2022 Advent of Fonts is an original creation of a serif-style font that places them in a somewhat unusual decorative fashion rather than the typical alignment to aid in reading.
Waterlily

Day 11 of the 2022 Advent of Fonts sees this creation with tall narrow lower case and large flowing upper case that combine together to quite lovely effect.
Calstone

An Advent of Fonts 2022 production for day 8. This font has been around on my drive for a while and I felt like it always needed a bit more attitude but there were no pixels left for it. On comparing it to the existing heavy fonts I have though it is different enough to stand apart and so here it is.
Comic Fans

Day 5 of my Advent of Fonts for 2022 sees my take on the font everyone loves to hate, Comic Sans, with this cute 8x8 pixel adaptation named, appropriately, Comic Fans!
Around

A 2022 escapee featuring massive characters that are trying to escape the confines of the 8x8 despite their thin rounded strokes. Corners and curves are over-plotted for a nice smooth look at high resolution or on CRT displays.
Cage

A late-2021 production originally intended for the 2021 Advent of Fonts but polished off in 2022.
Tipped

An original 2022 typeface for my Advent of Fonts inspired by thick felt-tip marker pens that stray into brush territory. Readability is good on LCD screens but some of the characters might be a bit too thick on a CRT display.
Raptor

Day 23 of my 2021 Advent of Fonts saw Raptor released.
Flamboyant

I love art-deco/Parisian 1920s typefaces and I didn't imagine I'd do another but on day 19 of my 2021 advent of fonts I did just that.
Vindicated

Day 14 of my 2021 Advent of Fonts saw Vindicated released based on the box art and advertising materials for the 1988 video game The Vindicator.
ZX Extendomatic

A 2021 adaptation of another of DJR's (David Jonathan Ross) fantastic typefaces - the LA-inspired Extendomatic for day 5 of my 2021 Advent of Fonts.
DistantTears

Day 7 in my 2021 Advent of Fonts saw me take on a new typeface in the style of the Far Cry logo.
Migrating from OpenTracing.NET to OpenTelemetry.NET
OpenTracing is an interesting project that allows for the collection of various trace sources to be correlated together to provide a timeline of activity that can span services for reporting in a central system. They were competing with OpenCenus but have now merged to form OpenTelemetry.
I was recently brought in as a consultant to help migrate an existing system that used OpenTracing in .NET that recorded trace data into Jaeger so that they might migrate to the latest OpenTelemetry libraries. I thought it would be useful to document what I learnt as the migration process is not particularly clear.
Developing a great SDK: Guidelines & Principles
A good SDK builds on the fundamentals of good software engineering but SDKs have additional requirements to consider.
Valley

A 2021 production with capitals originally inspired by DJR's Extendomatic but with a lower-case entirely of its own better suited to the constraints.
Phase Shifter

A 2021 production that took an angular approach to art-deco then morphed it into a magnetic character recognition style with a closed variant.
Segment8

A quick trip back to the 70s for a dip in the LCD & LED segment display technology that adorned watches, HiFi, and car audio equipment for a couple of decades.
Electromagnetic

A 2021 production combining the magnetic blobs with sharp angles of sci-fi to create something a little different.
Keytop

Sometimes when designing a font it can start to feel similar to another one. Normally a quick flip to my page and I'll find one that's either similar enough that I should make drastic changes or it's suitably different and I can continue. This one felt SO familiar but I couldn't find anything in my pages, nor in my _incomplete, or old rejected deletions.
PicoMag

A 2021 production taking the concept of a magnetic-character-recognition style and making it incredibly small. The bottom-heavy magnetic elements also give it a weight reminiscent of 80s chrome as well.
Hourglass

Up to this point all my sans fonts - well most of my fonts at all really - have used at most 6 vertical pixels for the x-height and typically only 5 pixels. This is because you need a row for the space and then another row for the dot on the i and then another for descenders.
Yaroze

A 2021 font constrained to 5-pixels wide with angles, attitude and overhanging curves and 26 of those dashing small-caps.
Runed

Runes predate our Latin based alphabet and directly informed and influenced it in many ways. Many of the earlier ones are not too recognizable but taking the Anglo-Saxon runes and a bunch of liberties.
Undead

A fresh 2021 production that draws inspiration from styles of lettering often used in dark and horror-themed environments.
Ultimatum

A hot-off-the-press 2021 production intended as a caps-only typeface based around the idea of a narrow oblique with very tight curves at the corners. A lower-case was added to complete the set, but it's the capitals and numerics that shine on this typeface, and a proportional renderer like FZX kicks it into gear.
Gemma

A 2021 production - yes, I'm still plotting in BASIN - that attempts to capture the feel of the Atari ST GEM/TOS high-res mode. The font itself can't be translated as it's way too tall being some 16 pixels high (yet only 8 wide).
Ricochet

A 2021 release of a type that's been sitting around a little while - from at least mid 2020.
Noted

So back in 2006 I created First Pass as a messy font that I would refine into a second pass but couldn't pull it off. Here I am in 2020 and I tried the experiment again from scratch this time drawing with the mouse in a single pass and then just moving a couple of pixels if needed.
ZX Eurostile

This 2020 BASIN production started as a discussion with Oli Wilkinson. He's working on a Red Dwarf game for the Spectrum. Anyone into fonts who watches Red Dwarf (probably all 4 of us) know, Eurostile is heavily-featured throughout the show, from signage to branded Jupiter Mining Corporation goods (in ALL CAPS).
Stepper

Stepper motors are fascinating devices and not just because they control floppy drives. Anyhow here's a 2020 BASIN production that has lots of steps all over the angles on a font.
Neoflow

This font was sitting around in this finished state for many months. Normally fonts stick around because they need work but this one was because I kept wanting it to fit with another font or wait until I had another font to go with it to form a family. Neither happened, it just is its own thing.
CannonFire

Occasionally when working on a font, I'll struggle with a specific glyph and push it in different ways to find something that works. Sometimes, the "very different" will lead to a font entirely on its own, which led to CannonFire.
Quickshot

The inspiration for this name, I believe, came from an advert for the QuickShot joystick. I can no longer find the source to confirm that.
Striker

An early 2020 release that has sat languishing for some time before publication. There's some similarity with Reynolds which has held it back but when you look at screenshots in both there's quite a subtle difference in the font size and choices in edges and letter forms.
Jam

I thought I'd covered most of the over-bold uneven styles I could in previous fonts but this one goes extreme in a top-heavy way and managed to pull off something a little different.
Anchovy

I designed this font in 2020 as a tribute to the DALEK font used in the 1964 Dr. Who tie-in Dalek Book. The font rose in popularity, with Caesar's Palace adopting it for their logo sometime later. The DALEK font did not include a lower-case, so I improvised there based on the cues in the upper case and numerics.
Enhancing content articles in Nuxt3
I've already covered reading time and generated excerpts for Nuxt3 content but there are still a couple more things you can do to enhance your content articles.
Star Maker

Olaf Stapledon's 1937 novel Star Maker sees the protagonist detach from his body and explore the infinite reaches of the universe across space and time.
Brashi

I was lucky enough to spend an afternoon on a practical introduction to Japanese calligraphy this year and the feel of those brushes have stuck with me.
Radial

Looking back at my childhood I can see early signs of my love for fonts. One such sign was the joy in using a pen to paint in the letters on the tyres of my dads car.
Advent of Fonts 2024
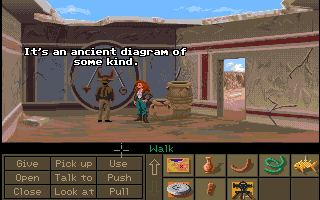
December 2024 sees the fourth year of my Advent of Fonts project where I published a 24-day advent calendar of 8x8 pixel on Mastodon.
Email form sender with AWS Lambda, Brevo & reCAPTCHA
In this article I'll show you how to use AWS Lambda to send an email with Brevo using their API while being protected by reCAPTCHA.
Quirky PC

Early PC video cards featured a familiar serif 8x8 font. Over the years different manufactures tweaked a few glyphs (ATI), made a serif version (Amstrad), or just plain stylized parts (AMI & Phoenix BIOS) all of which gave a slightly off-brand feel.
Echoes

A classy yet fun new typeface with a high contrast x-bar and large rounded edges.
Prophecy

Another new font this time with an old worn look that takes cues from ancient chiselled serif fonts while applying a hint of uncial hand lettering for a distinctly prophetic look!
Moffett

As a child of the 80s I loved the Airwolf TV show with its gorgeous helicopter (a modified Bell 222A), on-board computers, synth intro music and lone wolf hero.
Manic

When I saw a remake of Manic Miner called Manic Person under development on the Spectrum Computing forums I thought a custom font would polish off the look.
ZX Megascope

A 2023 adaptation of DJR's (David Jonathan Ross) fantastic Megascope font for April 2023.
A quick primer on floppy disks
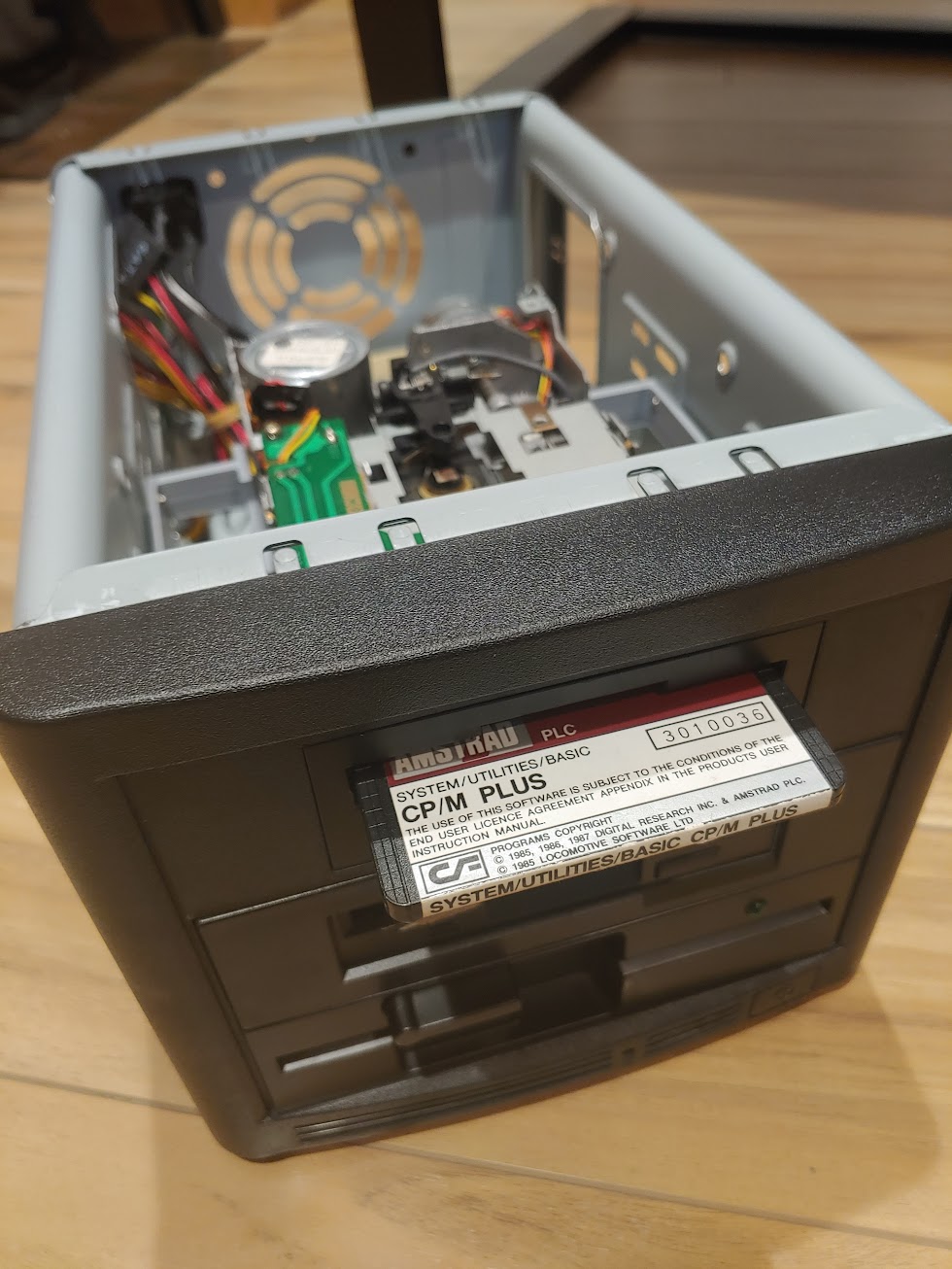
I've always been fascinated by floppy disks from the crazy stories of Steve Wozniak designing the Disk II controller using a handful of logic chips and carefully-timed software to the amazing tricks to create - and break - copy protection recently popularised by 4am.
Shakedown

This is day 23 - the penultimate day - of my 2022 Advent of Fonts calendar and we have a 1920s art deco font with some serious serifs and some not so serious for a little character.
Xcerpt

Advent of Font 2022's day 15 sees this italic serif handwritten font take to the stage. Italics are uncommon on handwriting styles but why not - after all I need to mix it up!
Rebound

A 2022 Advent of Fonts (Day 10) production that is sharp, bold, narrow and available in three weights.
Decor

An original font for day 7 of my 2022 Advent of Fonts calendar gifts. What we have here is a art-deco inspired font that drops the heavy single wide-stroke verticals traditional to fonts of this period and instead throws in some unexpected angles and some bar overshoot.
DockingBay

A December 2022 Advent of Fonts entry that pushes the capitals crossbar right up while introducing the odd serif-like flourish.
Software
Writing creating computer software is my passion in life and I've been lucky to work on some incredible projects and technologies over the years.
Kindness

One of the very few 2022 productions this is a tiny yet heavy and friendly font for use on space-constrained screens with a proportional renderer or where you just want a more unique look with plentiful letter-spacing.
Binnacle

A 2021 typeface inspired by the digital dash typography from the Peugeot 208-E although I've not been able to track down the exact font (it's not their rather nice custom house Peugeot font). It is a nice intersection between sharp lines and soft edges with nice wide glyphs.
Skid Row

This was day 22 in my 2021 Advent of Fonts and an adaptation of the font from Amiga cracking group Skid Row with their magnetic readable blob (MOCR) bubble-letters and extended it to a full set of lower-case letters.
Pristine

Day 16 of my 2021 Advent of Fonts saw Pristine released with a clean and a decayed version available.
Punch

Day 12 of my 2021 Advent of Fonts saw Punch released.
Prism

Day 4 in my 2021 Advent of Fonts saw the evolution of a distressed font that had been sitting around for a while. With some selective clean-up, angularization and limiting the distressing to the upper-case it came together nicely.
Founded

Day 3 in my 2021 Advent of Fonts and heavily based on the typography in the Apple TV adaptation of Isaac Asimov's Foundation series.
Sorcery

This mid-2021 typeface attempted to draw inspiration from the ZX Spectrum game Dragontorc by Steve Turner - a game I still have a copy of to this day but haven't touched in 20+ years.
Shinobi Extended

SEGA's 1987 ninja-themed horizontal-scrolling arcade game Shinobi is quite the classic with its sharp visuals and responsive play.
Gattars Fork

This mid 2021 design originally came out of a refinement to an as-yet-unpublished "Delta" series of fonts intended for futuristic space-ship signage. Somehow it started taking on a life of it's own picking up a few Inkscript flairs resulting in a font that's kind of hard to put your finger on.
Anvil

Anvil is a 2021 attempt to tackle the rustic, old-lettering but readable style made popular by many a Spectrum text adventure (without actually referencing it so as to avoid copying it).
Panda

A 2020-2021 production that started off as a CRT-specific font with exaggerated corners that would blur to provide some weight to them.
Tycho

A May 2021 production that hasn't been around too long based on the typography from the opening sequences of the phenomenal The Expanse TV-show.
Shipping breaking changes
Breaking changes are always work for your users. Work you are forcing them to do when they upgrade to your new version. They took a dependency on your library or software because it saved them time but now it's costing them time.
Watchface

An alternate take on Hourglass inspired this time by Hoefler & Co.'s Decimal typeface that captures the feel of many classical watch faces.
Authorized

A 2021 release that intersects the magnetic style of Computing 60s with a cut-out "stencil" style like No Step. A rounded-off version was added in 2022.
Prince

Jordan Mechner's phenomenal ground-breaking Prince of Persia needs no introduction. Even if you weren't around for the original, there's a good chance you've heard about it or played one of the many more-modern remakes and sequels.
Koncrete

Slab-serif fonts are my favorite family of fonts. From the incredibly gorgeous Guardian Egyptian to Rockwell and so many other slabs.
Kalopsia

A 2021 release that's been sat around since 2020 but it was time to let it free or send it to the big bit bucket in the sky. It escaped and perhaps it'll find a new home after all.
Area51

A 2021 effort that started from a discussion on Twitter about 51 column text on the Spectrum. While 64 column is quite common (3 pixels + space), 42 also quite common (5 pixels + space) and of course the default of 32 (8 pixels + space) a few people have created 51 column routines such as Micro Print 85 and Handywide.
Fountain

My first release of 2021 started off as an attempt to tackle the italic version of DJR's Extendomatic but I really wanted to do something different with the lower-case and so it took on it's own life.
Proforma

A 2020 attempt at creating a serious looking font without all the bother of squeezing in ascenders and descenders by using a small caps version I last tried with Localhost (which was designed to look quirky and not professional).
Quaked

I designed this font back in 2020 after seeing a screenshot of Star Quake - a game I very much enjoyed in my youth that had some nice early 8x8 typography.
LampLight

I'm a big fan of point 'n click adventures from my first experience with Monkey Island through Rex Nebula, Larry, Deponia, the incredible Broken Sword series as well as Simon the Sorcerer and modern takes such as Machinarium and Ron Gilbert's excellent Thimbleweed Park.
Tuner

A late 2020 BASIN production inspired by the aggressive typeface found on tuner cars and logos with cut-outs and an almost stencil bold look.
Halfling

A 2020 exploration into old-style hand-scripts such as you'd find in Lord of the Rings or Dungeons & Dragons.
Pinhead

This font started life as a conversion of the 1973 Wang 2200 minicomputer bitmap font. What surprised me was how it relied on the phosphor glow to give a nice effect despite being laden with gaps. This is really how all good fonts are designed - by modifying the input so the actual output on a real environment looks optimal.
Raven

This 2020 font takes inspiration from Defender of the Crown on the Amiga. I'd created a TrueType version of reproduction of that a while back.
Joshua

This is a 2020 port of my previously proportional FontStruct font WarGames-OpenCredits which is based on the opening credits of the 1983 hacker movie WarGames.
Inkscript

This early 2020 typeface has sat around for far too long in the incomplete. As is often the case it felt like it needed something at the time but looking at it later revealed it's already rather nice. Sometimes all it takes is fresh eyes and a reflection on the constraints.
Golden Air

Air America was an airline from 1950 through the 1970s that looked to all appearances to be a regular airline. In reality, it was covertly-owned by the US Government for CIA operations to access places the US Military could not. Employee William G. Sherman took courses in typography drafting and created an entire typeface based on their logo. Much later, his son reached out to the Internet in turning that typeface into a digital version, and Aaron Bell of Saja Typeworks took up the task.
Comical

Actual comic book typefaces are rather beautiful and Comic Sans - despite the name - doesn't really embrace the style instead opting for an unusual bendy lower case rather than the small caps angled lettering that accompanies gorgeous comic book art.
Tanzanite

The Amiga was the first machine I had where fonts were no longer constrained into 8x8 pixels. I played with FED on the Workbench 1.3 extras disk for quite a while!
Patrol

A new and original compact and aggressive font with cut-outs, unexpected serifs, and a full lower-case all available in three weights! If you were looking at my 2019 font Needlecast and thought "I wish this was more aggressive" then this is the font for you.
Scarlet

An all-original new bubbly font with a very low x-bar for added style and impact.
Generated Excerpts for Nuxt3 Content
Nuxt3 has been my stack of choice for a while now and it was time to port my site over from Nuxt2 - an exercise in itself I should blog about - but more concretely is the idea of excerpts.
Advent of Fonts 2023
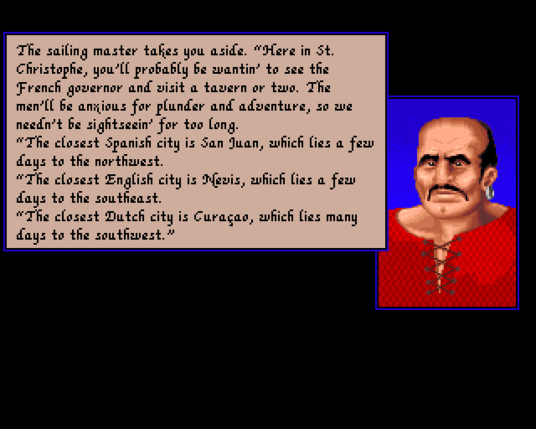
December 2023 saw the third year of my Advent of Fonts project where I published a 24-day advent calendar of 8x8 pixel on Mastodon.
Matinee

The 1920's art deco movement has inspired a lot of design and plenty of typography to go with it. Personally having already published SIX such 8x8 typefaces I wasn't sure I could do something new...
Track40

Here's to day 17 of my 2023 Advent of Fonts calendar - a tribute to floppy disks and all the magnetic glory with letter shaped a little like sectors on a track. I've always been fascinated by copy protection and the tricks you can pull off on a supposedly pure digital medium to stop it being duplicated.
Compass

This new font was inspired as being the counterpoint to my Protractor font that featured straight lines and harsh angles and instead takes broad rounded strokes as far as they can be squeezed in!
Undervolt

Thick heavy fonts with cut-outs and stylized edges aren't new in the world of typography gracing everything from TRON to AfterBurner and beyond but this new alternate take on the style with a real lower-case should bring polish to any space game.
A blog comment receiver for Cloudflare Workers
A number of years back I switched to a static site generator for damieng.com, firstly with Jekyll, and then with Nuxt when I wanted more flexibility. I've been happy with the results and the site is now faster, cheaper, more secure and easier for me to maintain.
The tricky part was allowing user comments without a third-party service like Disqus. I came up with a solution that has comments into markdown files just like the rest of the site content so they can be published as part of the build process.
Extracting files from Tatung Einstein disk images
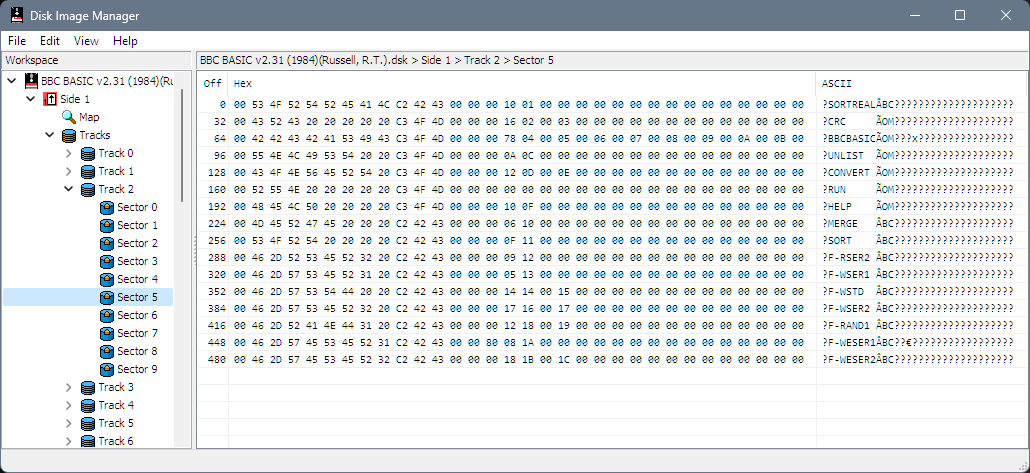
Recently Kevin Edwards got hold of some 3" disks containing source code to various old commercial games. He imaged them with the Kryoflux flux-level imager (Greaseweazle and FluxEngine are also good options). These tools produce highly accurate images of magnetic media that rips through copy protection and format concerns even allowing you to write the image back to disk with that in tact. This level of detail emits large files - 11.7MB for a single-sided Spectrum disk that normally holds 173KB is quite typical. 4KB data tracks happily turn into 215KB flux.
Adding reading time to Nuxt3 content
I've been using Nuxt2 quite a bit for my sites (including this one) and am now starting to use Nuxt3 for a few new ones and am finding the docs lacking in many places or confusing in others so hope to post a few more tips in the coming weeks.
Ident

My 2022 Advent of Fonts hits the final stretch with day 21 and this smart, oblique all-caps that features left-tick/serifs on the capitals only to give it a more kinetic feel. I'm quite happy how different this is from the other ~400 or so fonts in the ZX Origins collection so far.
Cyberwire

This font was originally drawn sometime in 2020 but never quite felt done. I dusted it off for the 2021 advent calendar but it still fit quite right.
Advent of Fonts 2022
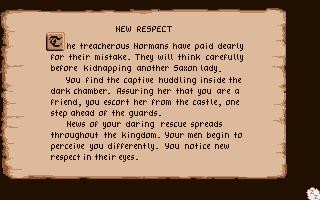
Through December 2022 I again produced a 24-day advent calendar of 8x8 pixel fonts this time primarily on Mastodon.
Outrunner

A 2022 Advent of Fonts production based on the numerics and capitals from the 1986 arcade classic Outrun. The original is a gorgeous gradiented colour font with an based on Yu Suzuki's previous game Space Harrier.
Advent of Fonts 2021

In December 2021 I tweeted a 24-day calendar of 8x8 pixel fonts.
Touch

A 2022 release that sat around for a while being experimented on in various forms and artistic stylings until settling on this after all sorts of experimentation with angles and slopes and then discarding them all.
Estimating JSON size
I've been working on a system that heavily uses message queuing (RabbitMQ via MassTransit specifically) and occasionally the system needs to deal with large object graphs that need to be processed different - either broken into smaller pieces of work or serialized to an external source and a pointer put into the message instead.
Xcelerant

Fresh off the pixel editor for 2022 is this hard oblique segment-inspired font.
Conveyance

Day 21 in my 2021 Advent of Fonts is Conveyance, a neat hand-written style typeface with tiny lower-case letters with towering ascenders.
Night in Tokyo

Day 16 of my 2021 Advent of Fonts saw the public release of a custom version of ZX Maverick I created for a text adventure I was working on called A Night in Tokyo.
Caprica

Day 9 of my 2021 Advent of Fonts saw Caprica released.
Using variable web fonts for perf
Webfonts are now ubiquitous across the web to the point where most of the big players even have their own typefaces and the web looks a lot better for it.
Quantra

This was the second font in my 2021 Advent of Fonts and a new angular take on sci-fi type.
Factor

Sacrificing the descender and re-working the glyphs that use them has opened up some extra possibilities I've explored a little with previous typefaces.
Privacy Policy
This privacy notice for Damien Guard ('we', 'us', or 'our'), describes how and why we might collect, store, use, and/or share ('process') your information when you use our services ('Services'), such as when you:
Widget

A mid-2021 design that goes for a lighter touch than my other serifs and instead allows for more whitespace. The complementary bold font uses the internal space to keep that relaxed external whitespace and providing a compressed look.
ZX Rustproof

A 2021 production that sees an adaptation of an adaptation - specifically this is an adaptation of Raymond Larabie's Rustproof Body - itself an adaptation of the distinctive lettering that adorned the back of everyone's favourite 1980s time machine - the DeLorean DMC-12.
ZX Extraordinaire

A 2021 adaptation of the gorgeous Extraordinaire variable font by type designer David Jonathan Ross. A semi-bold was added in 2024.
Lander

A 2021 alternative-take on the 80s-flavoured NASA-style typography. Unlike previous typefaces in this style (see Also Consider) this one takes a softer and more rounded approach on the upper-case and a more traditional humanist look on the lower-case.
No Step

A 2021 third-attempt at a stencil font this one directly inspired by the USAF technical manual and sporting caps for both upper and lower (the latter being just 1 pixel shorter) for a serious look.
Mac OS System 9 on Windows
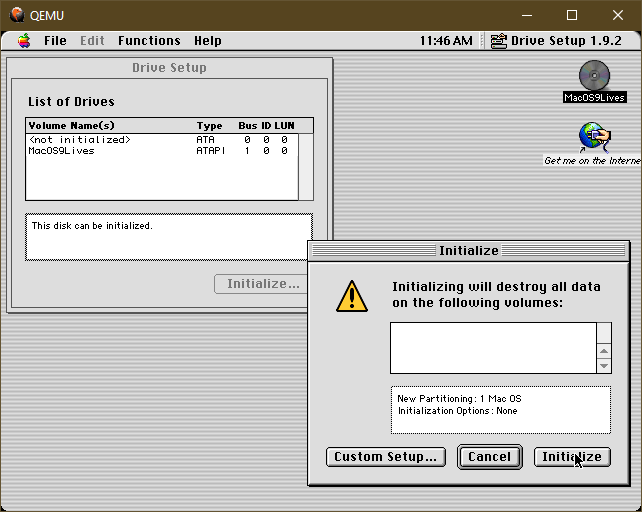
I'm often digging into old bitmap font and UX design out of curiosity - and someday hope to revive a lot of these fonts in more modern formats using a pipeline similar to that for ZX Origins so we can get all the usable fonts, screenshots etc. out of them.
Chit

I thought I was done with clean sans-serif designs having done a fair few. Imagine my surprise that when reaching for a sans-serif I found none of them quite fit the design I was looking for. ZX OCR-B came close but felt a bit too narrow in places and had those very distinguishable marks. I turned to Carton which had the wrong flourishes, Plotter was closer in flourish but was too wide and the condensed version too forced. I wanted something that would feel more proportional and go with the extra spacing than stretching everything out - something a bit more like ZX Palm but not so narrow.
Creating OR expressions in LINQ
As everybody who has read my blog before knows, I love LINQ and miss it when coding in other languages, so it's nice when I get a chance to use it again. When I come back to it with fresh eyes, I notice some things aren't as easy as they should be - and this time is no exception.
Quasar

A 2021 release of a design that had been sitting around for a while - at least since 2020 perhaps earlier - that never felt quite done or right. I eventually decided to just release the font anyway and in doing so tried to think of a name and decided that a spacey-name would be good which led to me remembering the Battlestar Galactica logo which has a similar theme.
Winterforge

Winterforge is a sharp, short, aggressive style drawn in 2021 that would work well for short runs of text in a fantasy adventure scenario.
Invasion

Another January 2021 release this time contrasting sharp angles and rounded curves available in two weights and with small-caps for lower case.
ZX Baveuse

A fresh 2021 production of Baveuse by prolific type designer Raymond Larabie. This type is probably his most well known after being used for the titles in the animated TV show Archer.
Beastly

Everyone has inspirations in their life that shape their idea of art, and Shadow of the Beast (2 specifically) was one of mine. Seeing that gorgeous artwork and the music that dynamically changed as you progressed through the area changed my opinion of what computers could do.
Torque

An end-of-year 2020 effort that started life as another attempt at the Protovision advert font from WarGames which I discovered is Serpentine. I did previously attempt it with my Reactor but I was never that happy with the oblique.
Syncwave

I must have encountered the joy of Synthwave music a year or two ago and it's quite heavily dominated what I listen to each day as I code.
JumpZone

An early 2020 effort that went through a few iterations having been originally inspired by the open contours of the Xenon II logo from the legendary game by The Bitmap Brothers.
Streetdoc

I think I started this font in 2019 and polished it off some time in 2020. It sat unfinished for quite some time as I was reluctant to publish fonts with no lowercase which somewhat constrains what can be done when you don't have adequate space for ascenders or descenders.
Valour

I designed this font in 2020 based on a few characters in the logo of the game Valorant.
From CircleCI to GitHub Actions for Jekyll publishing
I've been a big fan of static site generation since I switched from WordPress to Jekyll back in 2018. I'm also a big fan of learning new technologies as they come along, and now GitHub Actions are out in the wild; I thought this would be an opportunity to see how I can port my existing custom CircleCI build to Jekyll.
High Noon

This is my third attempt at a western wilderness woodblock font and it turned out quite well with the big blocky serifs. A bold version increases the vertical stroke which reduces the impact of the serif and removes some of the overshoot on horizontal strokes as there are, alas, no pixels left.
Shuriken

A mid-2020 font that needed a bit of a push over the line to make it work. Angular and sharp with overshoots and pointed serifs.
Polaris

In April 2020 Maxim from the Phantasy Star retranslation project reached out to see what could be done about the plain font in the Sega Master System game.