Changing type, the state pattern and LINQ to SQL
- 📅
- 📝 912 words
- 🕙 5 minutes
- 📦 .NET
- 🏷️ C#, design patterns, LINQ
- 💬 8 responses
A question I see from time-to-time on LINQ to SQL relates to changing an entity’s class.
C# and VB.NET don’t allow a class to change its type at run-time and LINQ to SQL specifically doesn’t provide a mechanism for changing the underlying discriminator for this reason.
Discarding the current object and creating a new one is fraught with issues. What do we do about existing references, unsaved data, established associations and caches?
Start with an example
Consider an abstract Account class with SavingsAccount and CurrentAccount sub-classes. Bank accounts don’t change type once created (in my experience) so that’s good so far.
When we get into processing and validation logic its tempting to create ClosedAccount and OpenAccount classes but what happens during execution when closing an account?
A further consideration is how exactly ClosedAccount and OpenAccount fit into the hierarchy given the single-inheritance limitation of C# and VB.NET.
Enter the State Pattern
The ever-resourceful Gang of Four describe the State Pattern as:
Allow an object to alter its behavior when its internal state changes. The object will appear to change its class.
Taking the bank accounts example and applying the state pattern gives:
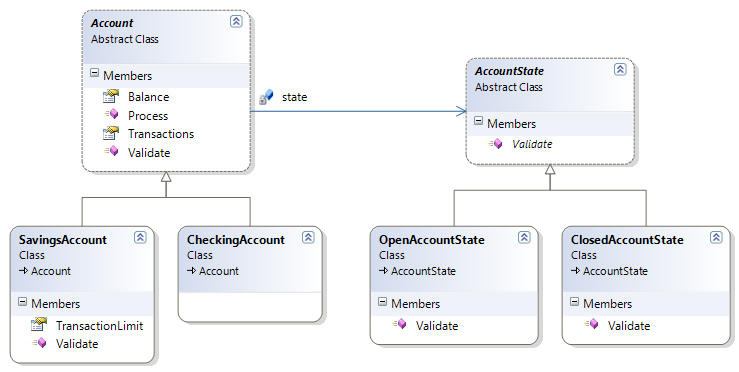
Account no longer needs to change type at run-time yet is still able to have clients call Validate and process Methods that end up in discrete methods as if inheritance had been used.
To achieve this we have:
- Created a State hierarchy that contains the logic we need to change at run-time
- Introduced a private member in Account that points to the current state instance
- Ensured the Account methods call the current state class via the private member
Because the state member is private and only accessed by Account itself we can happily create and dispose it as the conditions that affect the state change as much as we like without worrying about references to it.
This is best illustrated with code. Here rather than just calling the state’s validation logic there is a combination of core Account validation (balance), state validation (closed) and CheckingAccount validation (transaction limits):
public abstract class Account {
private AccountState state;
public virtual Status Validate(ITransaction tx) {
Status result = state.Validate(tx);
if (tx.Amount > Balance)
result.Add(TransactionFailures.InsufficientFunds);
return result;
}
}
public class SavingsAccount : Account {
public override Status Validate(ITransaction tx) {
Status result = base.Validate(tx);
if (Transactions.Count > TransactionLimit)
result.Add(TransactionFailures.TransactionLimitReached);
return result;
}
}
public class ClosedAccountState : AccountState {
public override Status Validate(ITransaction tx) {
return new Status(TransactionFailures.InvalidSourceAccount);
}
}
This is less complex than selectively replacing objects within our application at run-time and can bring additional benefits:
- Single responsibility principle – logic relating to a specific state has its own class to live in
- Favor composition over inheritance – inheritance for OpenSavingsAccount, ClosedCheckingAccount etc. is complex
Like all guidance, patterns and principles do not blindly follow these guidelines or patterns but consider how it affects and fits with your application. For this particular example it not only solves the problem but helps maintainability – at least at this simple stage. Once Validation becomes sufficiently complex it would likely move out entirely into a new set of orchestrated classes just for that.
With LINQ to SQL (and other mappers)
Moving this example into an object-relational mapper requires two – not unexpected – database-mapped properties.
- The inheritance discriminator (Type)
- A state indicator (Active)
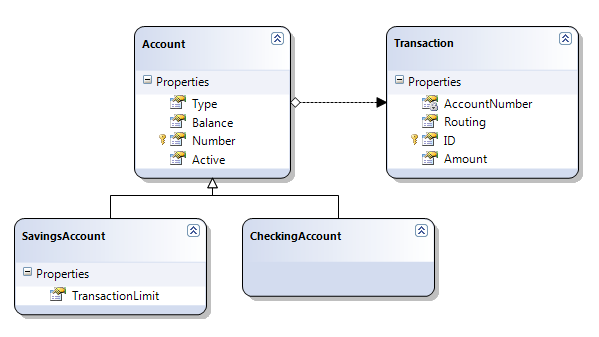
The only thing we need to ensure is the Account’s state member always refers to either a ClosedAccountState or OpenedAccountState depending upon the Active flag.
Given that LINQ to SQL code-generates the property for Active we could:
- Make Active private, wrap it in another property and set the state member when it changes and at initialization
- Make the state member a read-only property instead of an instance variable
The second works well here and given that AccountState is itself stateless (perhaps not the best choice of name) we can use a singleton to avoid multiple instances. The state instance variable in the Account class is replaced with:
private AccountState State {
get {
if (Active)
return OpenAccountState.Instance;
else
return ClosedAccountState.Instance;
}
}
The code continues to work and now changing the Active flag results in different behavior.
Best of all we still have the code in separate classes, no switch/case/if statements relating to validation or account types, a clean inheritance hierarchy and no running around trying to invalidate existing references.
Hitting the discriminator directly
There may be times when claims are made that a type has to change – perhaps data was entered incorrectly.
Before delving into the database or providing a tool to flip the underlying discriminator value consider:
- Does the new class interpret the same data in a different manner? Has a $1,000 credit limit just become a 1,000 transactions per-month limit?
- Would the object be valid in the new class? Did a ProposedCustomer just become ApprovedCustomer without a policy-enforced credit check?
- Are associations still honored? Are 300 unshipped orders for a GameProduct still honored for a BookProduct?
If in doubt don’t do it.
An inconsistent database bleeding through your application isn’t good for anyone and will take a lot longer to sort out than setting up a new entity.
[)amien
8 responses to Changing type, the state pattern and LINQ to SQL
While I think it's true that its a smell if entities in a LOB application change their type, I think it's actually pretty common in a knowledge manipulation/creative application.
I've lost count of the times I've wanted to change a geometry to an image or a struct to a class or similar choices.
The state pattern can work here, although it primarily concerns itself with behavior. In these knowledge-based apps, data frequently changes as well, so you need to have some sort of exchange mechanism where you can move the subset of data that is applicable across. Also the object then needs to change its set of attributes for later manipulation. That implies that you have a dynamic enough typing mechanism that the aggregated state object can appear to project its properties up to the parent object for anyone that cares - otherwise you're breaking your encapsulation. I've never tried mapping that to a RDBMS - interesting topic.
Unfortunately business rules these days are very complex and the database is unsuited to advanced programming environments/language/constructs that would be required not to mention the issues and costs involved in scaling those out compared to putting the logic in business layers.
It's the same server-vs-client argument that has caused the swings back and forth over the last x number of years.
It's still too bad that most ORM folk come from the Coding world rather than the Database world. For example,
Hitting the discriminator directly
has 3 cases which you worry about. As one from the Database world, the answer is straightforward: these are constraints, stored in the catalog as plain text, and can be read from said catalog either at compile time or run time or both and turned into local Edit Checks as desired. There are products in existence which do so.
The advantage: by having data and its constraints of record stored together in the Database Engine leaves no room for execution error due to sync-ing among however many tiers one chooses to use. All constraints which are applied in a tier are validated against the catalog. This is the whole point of storing to a RDBMS; storing to bespoke files (xml or VSAM or ...) will always be faster. Less power for coders, but too bad.
I just did some looking elsewhere, and it seems that LINQ is not designed to generate validation from the catalog, beyond simple stuff. Really too bad; it would be killer if it did.
In the Database world, the Prime Directive: a row is a business rule. Date wrote a book about that, although it is obvious.
The concept of making the state pattern a first-class citizen in an ORM is an interesting one and if type discriminators are supported then why not?
I assume by session you mean the data gateway and identity map? If so, the problem there is when there are multiple references to a single entity object that is now taking part in a unit of work.
It would be possible to create a copy of the entities as they participate to ensure changes are isolated and then apply those back to the original entity instance once committed. Having to specifically enroll entities in the unit of work and know they won't propagate out would be a bit of a mind-shift for many but worth it in my opinion.
When I was writing a custom-ORM some time ago, I had an identical concept. Boolean flags (like "Active") are the uncommon, simple case. The more general case in an ORM model is that you really have to carefully consider every discriminator value in your types, as to whether it is constant over the lifetime of an instance, or it can change. If it is constant, then inheritance is the simpler pattern. If it is not, then States are a better pattern.
In my case, I was able to focus considerable effort on making States into a first-class citizen in the ORM. This enabled things like exposing optimized collections like "Customer.ActiveAccounts", and also having the change of the discriminator automatically move the account from Customer.ActiveAccounts to Customer.NonActiveAccounts.
A more realistic example is that you have a status-discriminator with multiple possible values, and that status is stored in a separate table than the main account data. In addition, you have some other discriminator flags. It can easily be that "Active" is a broad concept which must be derived from multiple discriminators. For example, Active is true when "open-flag=true and status!=suspended". Consider the value of an automatically available (not hand-coded) Customer.ActiveAccounts in this context - it is a very low-friction way of working with data.
My point is that the State pattern should be a first-class citizen in any ORM model. Without it, you have a fair amount of friction (for example having to manually code the simple boolean logic for my "Active" example) and performance issues (because the ORM cannot auto-generate a query for "Active" accounts the way it could if it were a "Type" discriminator).
Score -1 for every commercial and open-source ORM I've encountered so far. Does anyone know if there an ORM that even knows about States? While I'm here, does anyone know if there is one that does not couple the orthogonal concepts of Session and Unit of Work? (You can probably tell that I have a very low opinion of the ORMs I have encountered).
I'd argue that account types are not universally fundamental. The distinction between 'checking' and 'savings' is an American thing, in that there are Federal laws that say you can't pay interest on a checking account. Not so in other countries. In the UK a 'Current' account isn't anywhere near as restricted a definition as a 'Checking' account, and the line between 'Current' and 'Savings' is regularly very blurry.
The terms of bank accounts tends to change from time to time, interest rates etc. but I've never had a bank account change it's fundamental type...
Funnily enough I've had my bank accounts change type many times, due to the bank running promotional new types of account, time-limited sign-ups that then revert to other types etc. But obviously your use of the State pattern could equally be used for that (or the Strategy pattern). The GoF is one book that just keeps on giving.
There's actually not a lot of practical difference between the State and Strategy patterns. The only real difference is that Strategy tends to hold no state of its own, it's a pure functional object and can therefore be shared between stateful instances, swapped in and out more freely, while the State pattern is strongly associated with parent stateful structures.